Let's get stuck in
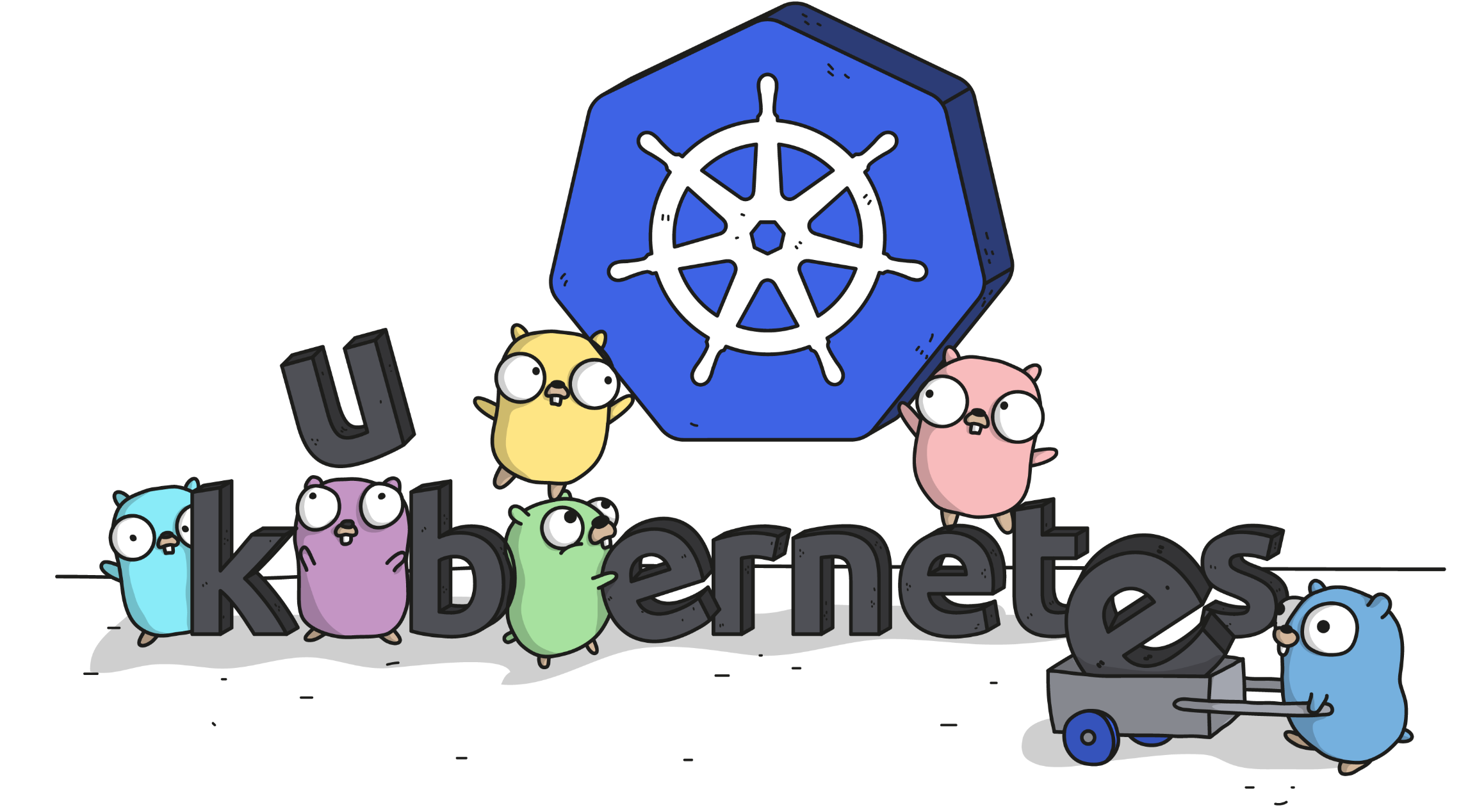
Kubernetes is only about 4 years old, but already has seen adoption that rivals the levels of cloud
Kubernetes is huge, I mean REALLY huge! As of 2018 it is fair to say that Kubernetes ranks amongst the top 5 most sought after skills for technology companies (Along with AWS, Python, Docker and React).
To be frank, it doesn’t really matter if you are a Developer, Tester, DevOps or SysAdmin - Kubernetes skills are worth its weight in gold. The reason is that Kubernetes encompasses several bleeding edge Infrastructure and Software Development concepts:
Explanation: Kubernetes is an open-source container-orchestration system for automating deployment, scaling and management of containerized applications. What this means is it works as a kind of hub for Pods which in turn comprise of containers (most commonly docker containers), allowing you perform management on mass , such as spinning up 20 pods when traffic demand increases. Inside each container is a stripped down bloat free Linux OS which has your application installed. The reason for this is it makes software development modular, moves away from big monolithic services and can be easily migrated too and from the Cloud.
In this tutorial I am going to take you through the steps needed to install MiniKube which is our local Kubernetes cluster manager, write a simple NodeJs application, wrap that up in a Docker container and use Kubernetes to deploy, manage and load balance our container.
Warning : It seems like there is a lot of code, but most are one line commands and I explain each step on the way. Don’t be put off, ultimately much of this can eventually be done in a YAML file, but learning how to use the kubectl interface is the first step in becoming a kubernetes master!
Installing MiniKube
In this walkthrough we will be using minkube to create a local cluster on your machine, these steps are for use with Mac operating system, if you are using another system the steps are a little bit different. Please google "Minikube installation guide" it will be the first hit
Install via Mac Brew
brew cask install minikube
Next lets install the kubernetes CLI, this will allow us to interarct with the cluster directly via the command line.
Install Kubernetes CLI
brew install kubernetes-cli
Ensure you have docker up and running, if you don't have it installed I would recommend installing 'docker for mac' that is the most stable popular instance.
Check Docker Up and Running
docker images
Now its time to start your Minikube session.
Starting Minkube
minikube start
Lets set the Minikube context. The context is what determines which cluster kubectl is interacting with. You can see all your available contexts in the ~/.kube/config file. We will also run another step to check cluster-info after just to ensure kubectl is configured correctly
Setting the Context
kubectl config use-context minikube
kubectl cluster-info
Finally in this section you can check the dashboard, (get in the habbit of running this command to keep a view of what is going on with your cluster)
View the Dashboard
minikube dashboard
Building your Node Application
Of course as the purpose of this tutorial is to walkthrough kubernetes, we won't spend too much time on our Node applicaiton. First off, you will need to install node and npm on your mac, once done come back to this section to create a very simple app.
It may be hard to appreciate the utility of Kubernetes running a simple app like this, but try to imagine the potential of what this could be. For example, this could be a node and react front end applicaiton, with a persistence database and Java backend - which could quickly scale in complexity. Ultimately the approach for running these applications in pods remains the same as our basic app we are going to build now.
Copy the code below into a file and save as server.js
server.js
var http = require('http');
var handleRequest = function(request, response) {
console.log('Received request for URL: ' + request.url);
response.writeHead(200);
response.end('Kubernetes Rocks!' );
};
var www = http.createServer(handleRequest);
www.listen(8080);
Now lets run our application, using the command below (make sure you are in the same directory as the server.js file for this whole tutorial. Once you have run the command you should be able to see our 'Kubernetes Rocks!' message at http://localhost:8080/
Run your application
node server.js
Create a Docker container image
Next we want to create our Dockerfile that basically acts as a template for building docker images. When you run a docker build command, it picks up the Dockerfile from that directory and builds the image. This Docker container image we are building extends the server.js image
Dockerfile
FROM node:6.14.2
EXPOSE 8080
COPY server.js .
CMD node server.js
Normally when we kick off Docker build commands it stores the image in your machines docker registry. However since Minikube also has a Docker registry we need to point to that one. Note If you need to roll back and finish this tutorial early, run the following comamnd eval $(minikube docker-env -u).
Point to Minikube docker registry
eval $(minikube docker-env)
Now lets build our docker image, don't forget to copy the trailing dot below or it won't work.
Build Docker Image
docker build -t myfirstpod:v1 .
Finally lets check our Minikube docker registry is up and running by connecting and having a look at our images.
Check Minikube Docker Registry
minikube ssh docker images
You should see your image in the list.
Create a deployment
For clarify a Kubernetes Pod is a collection of containers, in this case we are just using one Docker container, but wrapping them up in a deployment pod makes it more logically efficient. If a cointaier/pod was to fail or terminate - Kubernetes automatically spins up a new one! Note in the instructions below, we specify in the flag to not pull from native docker registry - this means we are sticking to using our Minikube registry
Build myfirstpod Deployment
kubectl run myfirstpod --image=myfirstpod:v1 --port=8080 --image-pull-policy=Never
View Deployment
kubectl get deployments
View Pod
kubectl get pods
Create a Service
We are getting close to the end now, so good job for getting this far! Creating a Service is the next logical step after creating a deployment. First off we need to open up our ports so the service can be viewed externally
Expose Deployment
kubectl expose deployment myfirstpod --type=LoadBalancer
View Service
kubectl get services
Finally lets actually view the output of our service by running the command below. This automatically opens a browser using a local ip address to serve content.
Start service
minikube service myfirstpod
Remember the get kubectl get pods command from earlier, use that pod ID to drive the command below to view the logs of your application.
View Pod Logs
kubectl logs YOUR-POD-NAME
FANTASTIC work!! You now are running docker containers in Kubernetes load balanced Pods! Do you remember the command from earlier to view the dashboard? Try running that again, have a look at what is changed.
Update our App
So what happens if the developers decide to make changes, add a new version of the app for deployment - No problem! This is what Docker and Kubernetes are designed for. Lets edit our server.js file, and change the message as below.
Amend Server.js file
response.end('Kubernetes REALLY Rocks!!');
Build a new version of your image
docker build -t myfirstpod:v2 .
Update the image of your Deployment
kubectl set image deployment/myfirstpod myfirstpod=myfirstpod:v2
Finally lets run the app again, and view the message to ensure it has updated (and in fact our new version is running).
Run the Service
minikube service myfirstpod
Clearing down and cleaning up
Seriously you have done very very well to get this far, give yourself a pat on the back! Now its time to clear up our resources, and tear down our Kubernetes environment. You may want to retain the images for later - but I always find doing a full delete after a tutorial is important if you want to save space.
Delete Service and Deployment
kubectl delete service myfirstpod
kubectl delete deployment myfirstpod
Remove Docker image
docker rmi myfirstpod:v1 myfirstpod:v2 -f
Stop minikube and remove pointer
minikube stop
eval $(minikube docker-env -u)
Delete Minikube
minikube delete
Where Next?
I hope that by going through this tutorial you were able to see the utility and power of Kubernetes. Although the journey doesn’t stop here, what if you wanted to automatically provision Pods to with application ready Front end, Back end services, roll out DataBases and replicate them as read traffic increases or add storage dynamically.
These features and much much more are part of Kubernetes IAAS feature, by designing YAML files to act as a template for our entire infrastructure, we can have a ready to roll out end to end service delivered within minutes.
For us however, take time to digest what we did, try and re-run the steps and see what sticks in the memory. Take a break and once you are ready to jump back into the fray - start looking at working with Kubernetes YAML declarative files.
Adam McMurchie
07/October/2018
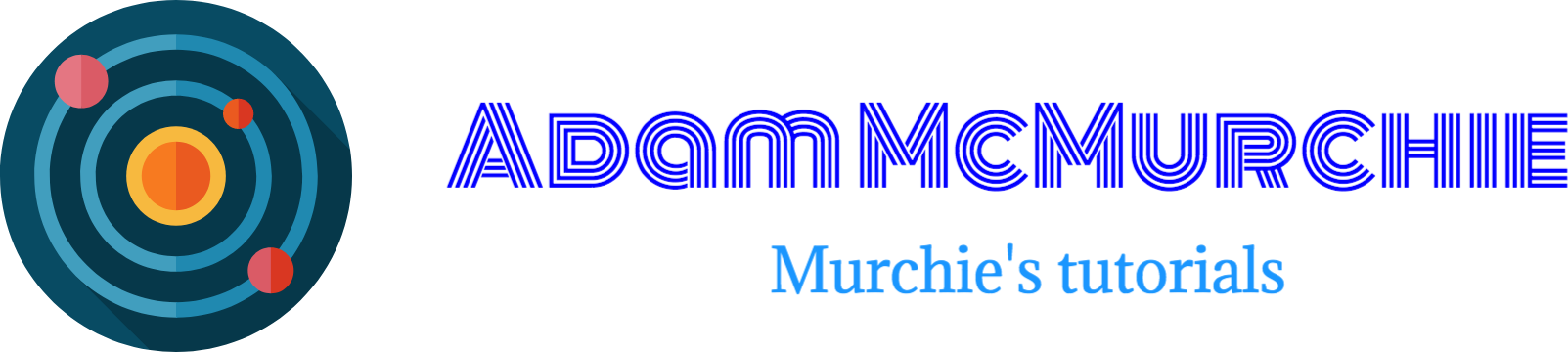